Going 3D with Trois.js and Vue 3
Trois.js allows you to bring the 3D magic of Three.js into the modern world of Vite.js ⚡️ and Vue3 💚.
In this article, we are going to learn the basics. I will assume you have some notion about 3D before continuing. You can find the complete repo here.
yarn create vite troisjs-example --template vue
Add Trois.js to the project:
yarn add three@0.127 troisjs
You can install it as a plugin:
import { createApp } from 'vue';
import { TroisJSVuePlugin } from 'troisjs';
const app = createApp(App);
app.use(TroisJSVuePlugin);
Or importing the resources directly into your component (This enable tree shaking and typescript support):
import { defineComponent } from 'vue';
import { Box, Camera, LambertMaterial, PointLight, Renderer, Scene } from 'troisjs';
export default defineComponent({
components: { Box, Camera, Renderer, Scene, PointLight, LambertMaterial },
});
# Core components
The more basic example const of 3 core components:
- Render: enables to perform 3D rendering in an HTML canvas.
- Camera: that uses perspective projection. This projection mode is designed to mimic the way the human eye sees.
- Scene: Scenes allow you to set up what and where is to be rendered by three.js. This is where you place objects, lights and cameras.
<script lang="ts">
import {
PointLight,
Box,
Camera,
Renderer,
Scene,
LambertMaterial,
} from 'troisjs';
import { defineComponent } from 'vue';
export default defineComponent({
components: { Box, Camera, Renderer, Scene, PointLight, LambertMaterial },
});
</script>
<template>
<Renderer resize="window" orbit-ctrl ref="renderer">
<Camera :position="{ z: 10 }" />
<Scene background="#4DBA87">
<PointLight :position="{ y: 50, z: 50 }" />
<Box ref="box">
<LambertMaterial />
</Box>
</Scene>
</Renderer>
</template>
To break down a little bit the code above, we set a Renderer
component as a wrapper, we add resize="window"
to make the canvas responsive, orbit-ctrl
allows you to use the mouse to change the camera position (Initially on z: 10
) dynamically, so you can rotate the object as you wish.
Inside Scene
, we have PointLight
which is a component that enables a light that gets emitted from a single point in all directions (think of it as a bare lightbulb 💡) and a Box
mesh with a LambertMaterial
inside as a slot (non-shiny surface without specular highlights).
Use yarn dev
to see the result on the browser:
# Render loop
Trois.js uses the requestAnimationFrame
loop under the hood to render the scene. Usually runs at 60fps
.
Let's use it to give some life to the box once the scene is rendered, first of all, make sure you are referencing the renderer and the box with template refs:
<script lang="ts">
import {
PointLight,
Box,
Camera,
Renderer,
Scene,
LambertMaterial,
} from 'troisjs';
import { defineComponent, onMounted, ref } from 'vue';
export default defineComponent({
setup() {
const renderer = ref(null);
const box = ref(null);
onMounted(() => {
renderer?.value?.onBeforeRender(() => {
box.value.mesh.rotation.x += 0.01;
});
})
return {
renderer,
box
}
}
};
</script>
Once we mount
our host component, we will listen to the onBeforeRender
event on the renderer
object instance and wait for it to start rotating the box by accessing the rotation
property inside the mesh
.
# Conclusion
Trois.js allows you to quickly set up a 3D project with top-notch modern frontend technologies. It's pretty performant and easy to use.
- To learn how to use more features, check out trois.js features.
- For the extended video tutorial of this article, check Going 3D with Trois.js (Three.js + Vite)
Happy coding!
Related Articles
The new Provide and Inject in Vue 3
Getting stuck into the prop drilling? Learn how provide/inject can make your components more flexible and independent in this short tutorial.
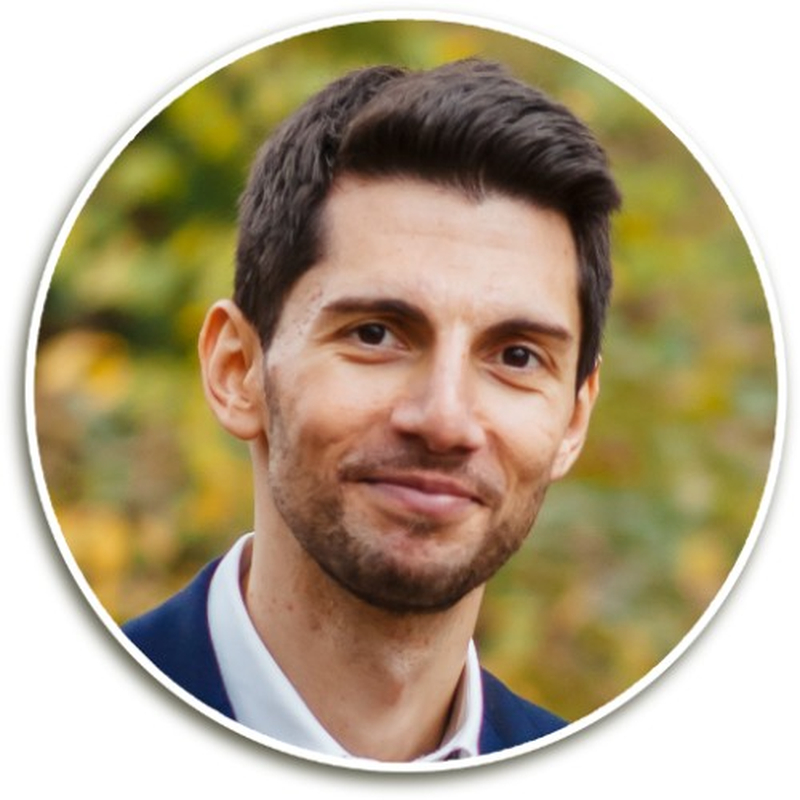
Jul 18, 2022
The 101 guide to Script Setup in Vue 3
Don't you know about Script Setup yet? Check out this short article now and learn the nicest way to define a Vue component right now!
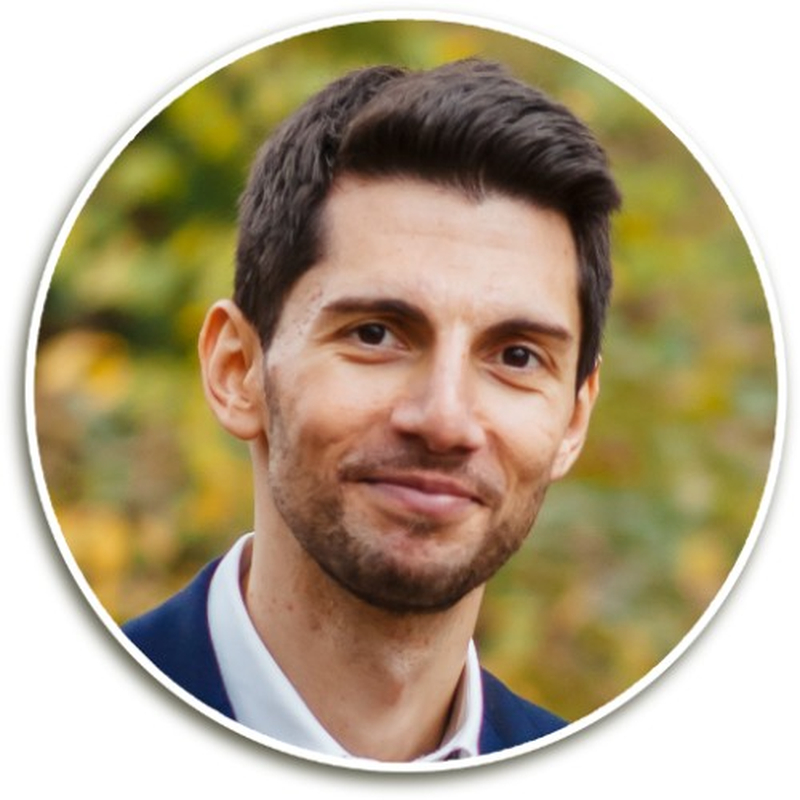
Jun 20, 2022
Use Composition API to easily handle API requests in Vue.js
Not sure how to organize your API client in Vue.js? Learn this simple technique on how to do it using the new Composition API and make it easy.

Jul 6, 2020
Sponsors
VueDose is proudly supported by its sponsors. If you enjoy it, consider supporting it to ensure the project maintainability.