Auto Load Vuejs Components in Nuxt
With Nuxt components you can auto import your components really easily and even comes with support for dynamic imports otherwise known as lazy loading. That means you can just add your component in the template without having to add it to the script tag. This makes development much faster.
# How does it work?
This module parses your template and automatically includes the component in the file where you are using it such as a page, layout or even a component. Because Nuxt.js uses automatic code splitting to split your pages by default this module works perfect as it will only contain the components that are used on that page. Also, if you use a component in more than 2 pages, Nuxt.js will automatically create a shared chunk for them thanks to the magic of webpack.
# Installation
If you are using Nuxt v2.13+ then this module is included by default but it is not activated by default so you don't have to install it but you do have to set components to true in your nuxt config file.
export default {
components: true
}
OR
If you are using Nuxt 2.10+ then you will need to install the module.
yarn add --dev @nuxt/components # or npm install --save-dev @nuxt/components
Then you need to add the @nuxt/components to your buildModules section of your nuxt.config.js file.
export default {
buildModules: [
'@nuxt/components'
]
}
# Auto Import your components
Once you have the module activated or installed you can now simply add your components in your template. The module will read the components directory and automatically look for the component in there.
- Create your components
components/
TheHeader.vue
TheFooter.vue
- Use your components in any .vue file (components, pages or layouts) without having to add a script tag or an import.
<template>
<TheHeader /> <!-- or <the-header /> -->
<TheFooter /> <!-- or <the-footer /> -->
</template>
# Dynamic Imports
You can also dynamically import your components or lazy load your components by prefixing the world Lazy in your templates.
<template>
<TheHeader />
<LazyTheFooter /> <!-- lazy loaded -->
</template>
This is great for when you want to load a component only on a click event for example. Here we have a component called MountainsList in our components folder and we add it to our template. We only show it if show is true. The button will appear if show is false and on click it will call the showList method which will change the value of show to true and this will therefore lazy load your component.
<template>
<div>
<h1>Mountains</h1>
<LazyMountainsList v-if="show" />
<button v-if="!show" @click="showList">Show List</button>
</div>
</template>
<script>
export default {
data () {
return {
show: false
}
},
methods: {
showList() {
this.show = true
},
}
}
</script>
You can check how this works by opening your browsers network tab and clicking on JS. Then if you refresh the page you will see the javaScripts that are loaded and when you click the button you will then see the MountainsList.js is loaded.
For more options or to see a live CodeSandBox check out this link
ℹ️ Nuxt components will not work with dynamic components. For these components you will have to use the script tag to import your component.
<component :is="componentName" />
Related Articles
How to structure a Vue.js app using Atomic Design and TailwindCSS
Unsure about how to organize your Vue.js components? Learn in this tutorial how to do it using Atomic Design methodology. Examples and demos included!

Jun 16, 2020
The most modern Pie Chart component using CSS Conic Gradient and Vue.js
Build a Pie Chart component using one of the modern CSS features Conic Gradient
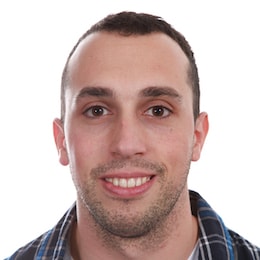
Oct 21, 2019
The most modern Carousel component using CSS Scroll Snap and Vue.js
Build a Carousel by using one of the latest CSS features called scroll-snap and bundle it into a Vue.js component
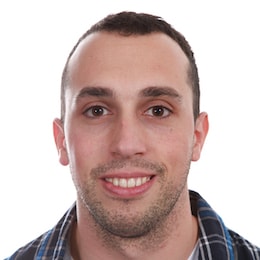
Oct 6, 2019
Sponsors
VueDose is proudly supported by its sponsors. If you enjoy it, consider supporting it to ensure the project maintainability.